如何在绘图中添加不同的颜色、线条样式、渐变、图案、阴影
1.色彩 Colors
1.1设置图形填充颜色 fillStyle = color
1.2设置图形轮廓颜色 strokeStyle = color
color可以是表示 CSS 颜色值的字符串,渐变对象或者图案对象。
fillStyle
1 | function draw() { |
效果如下:
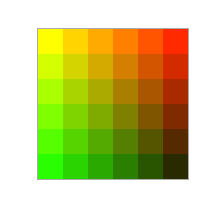
strokeStyle
1 | function draw() { |
效果如下:
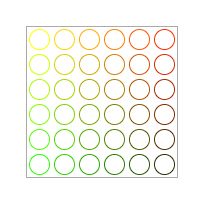
2.透明度 Transparency
除了可以绘制实色图形,我们还可以用 canvas 来绘制半透明的图形。通过设置 globalAlpha 属性或者使用一个半透明颜色作为轮廓或填充的样式。
2.1 通过globalAlpha设置全局透明度 globalAlpha = transparencyValue
globalAlpha属性影响到 canvas 里所有图形的透明度,有效的值范围是 0.0 (完全透明)到 1.0(完全不透明),默认是 1.0。
2.2 通过CSS3颜色值设置透明度
1 | // 指定透明颜色,用于描边和填充样式 |
globalAlpha
1 | function draw() { |
效果如下:
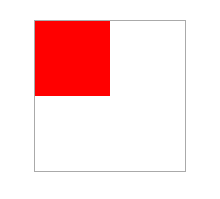
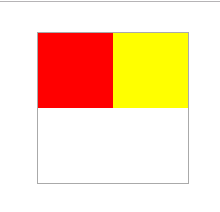
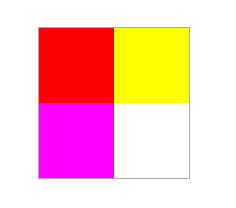
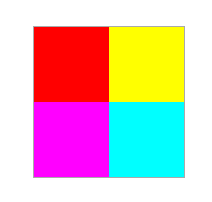
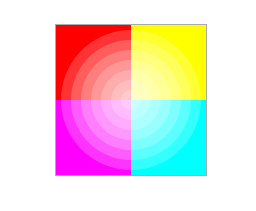
注意:先画图,还是先设置透明度ctx.globalAlpha = 0.2,所得的图形透明度有差异
1 | function draw() { |
效果如下:
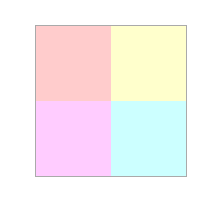
CSS3颜色值
1 | function draw() { |
效果如下:
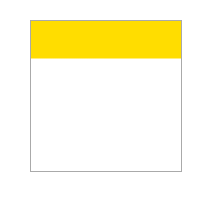
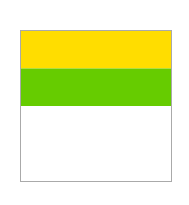
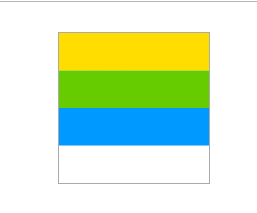
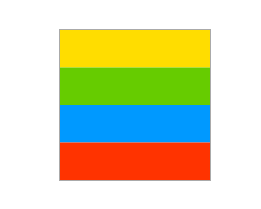
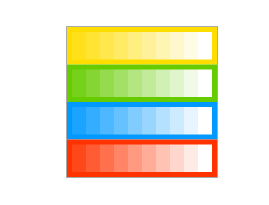
3.线型 Line styles
3.1设置线条宽度 lineWidth = value
线宽是指给定路径的中心到两边的粗细。换句话说就是在路径的两边各绘制线宽的一半.
所有宽度为奇数的线并不能精确呈现,这就是因为路径的定位问题。
1 | function draw() { |
3.2设置线条末端样式 lineCap = value
3.3设置线条与线条结合处样式 lineJoin = value
3.4限制当两条线相交时交接处最大长度 miterLimit = value
所谓交接处长度(斜接长度)是指线条交接处内角顶点到外角顶点的长度。
3.5返回当前虚线样式 getLineDash()
3.6设置虚线样式的起始偏移量 lineDashOffset = value
4.渐变 Gradients
用createLinearGradient、createRadialGradient新建一个 canvasGradient 对象
4.1 createLinearGradient(x1,y1,x2,y2)
createLinearGradient 方法接受 4 个参数,表示渐变的起点 (x1,y1) 与终点 (x2,y2)。
1 | var lineargradient = ctx.createLinearGradient(0,0,50,50) |
4.2 createRadialGradient(x1,y1,r1,x2,y2,r2)
createRadialGradient 方法接受 6 个参数,前三个定义一个以 (x1,y1) 为原点,半径为 r1 的圆,后三个参数则定义另一个以 (x2,y2) 为原点,半径为 r2 的圆。
1 | var radialgradient = ctx.createRadialGradient(50,50,0,50,50,100) |
4.3 addColorStop(position,color)
创建出 canvasGradient 对象后,我们就可以用 addColorStop 方法给它上色了。
- position 参数必须是一个 0.0 与 1.0 之间的数值,表示渐变中颜色所在的相对位置。0.5 表示颜色会出现在正中间
- color 参数必须是一个有效的 CSS 颜色值
1
2
3var lineargradient = ctx.createLinearGradient(0,0,150,150)
lineargradient.addColorStop(0,'#fff');
lineargradient.addColorStop(1,'#000');
createLinearGradient例子
1 | function draw() { |
效果如下:
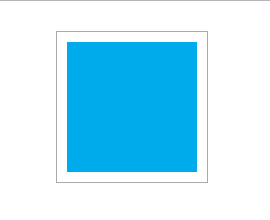
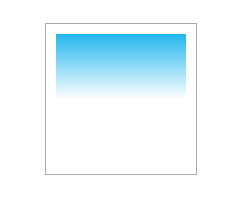
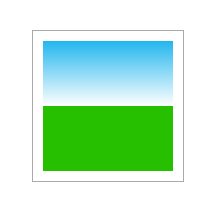
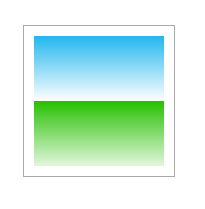
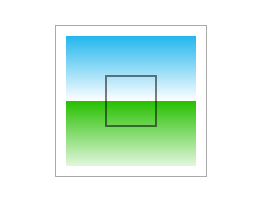
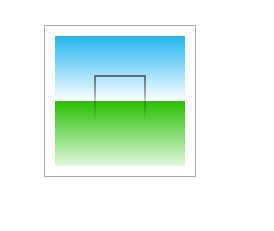
createRadialGradient例子
1 | function draw() { |
效果如下:
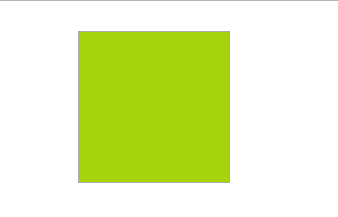
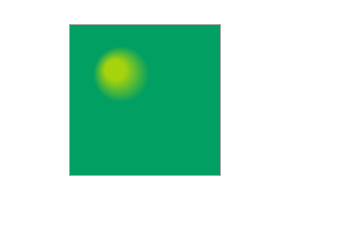
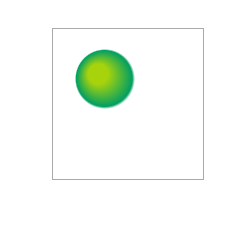
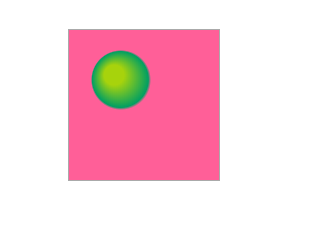
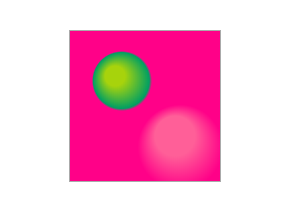
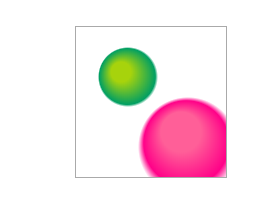
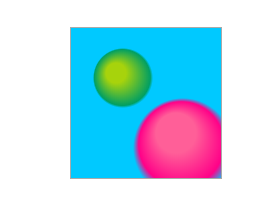
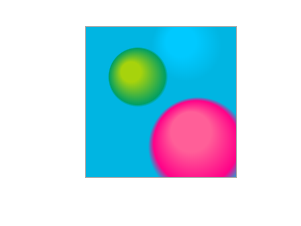
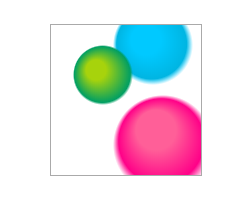
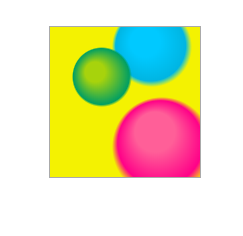
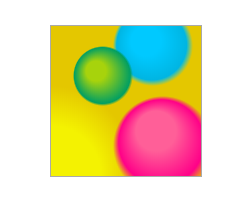
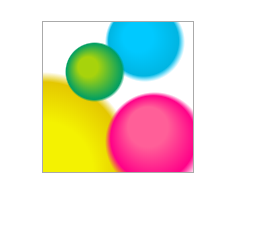
5.图案样式 Patterns
5.1 createPattern(image,type)
- Image 可以是一个 Image 对象的引用,或者另一个 canvas 对象。
- Type 必须是下面的字符串值之一:repeat,repeat-x,repeat-y 和 no-repeat。
1 | function draw() { |
效果如下:
repeat
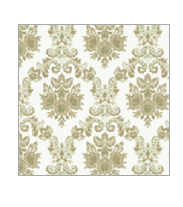
repeat-x
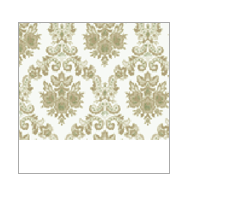
repeat-y
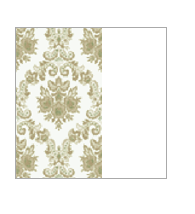
no-repeat
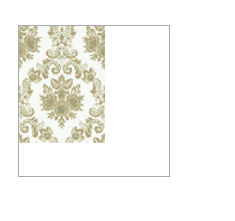
6.阴影 Shadows
6.1 设定阴影在x轴的延伸距离 shadowOffsetX = float
负值表示阴影会往上或左延伸,正值则表示会往下或右延伸,它们默认都为 0。
6.1 设定阴影在y轴的延伸距离 shadowOffsetY = float
负值表示阴影会往上或左延伸,正值则表示会往下或右延伸,它们默认都为 0。
6.1 设定阴影的模糊程度 shadowBlur = float
其数值并不跟像素数量挂钩
6.1 设定阴影的颜色效果 shadowColor = color
标准的 CSS 颜色值,用于设定阴影颜色效果,默认是全透明的黑色。
1 | function draw() { |
效果如下:
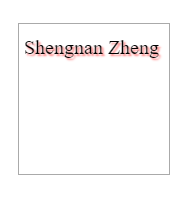
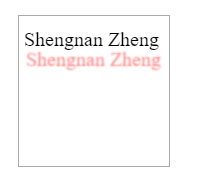
7.Canvas填充规则 Canvas fill rules
当我们用到 fill(或者 clip和isPointinPath )你可以选择一个填充规则
“nonzero”: 非零缠绕规则, 默认值.
“evenodd”: 奇偶缠绕规则。
1 | function draw() { |
效果如下:
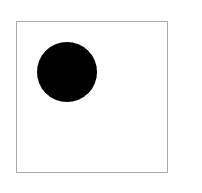
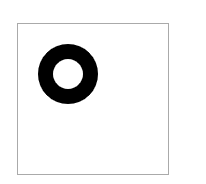